Interacting with web services and APIs is a vital skill for developers, system administrators, and anyone who needs to retrieve data from the internet. One of the most versatile and powerful tools for this purpose is cURL. In this guide, we’ll focus on making GET requests using cURL and use the Free Photos API as an example. Remember, the API itself is provided solely for demonstration purposes—the main focus here is on mastering GET requests with cURL.
Table of Contents
- Introduction to cURL and GET Requests
- Why Use cURL for GET Requests?
- Anatomy of a cURL GET Request
- Overview of the Free Photos API
- Using cURL: Basic Examples
- Advanced cURL Options
- Troubleshooting Common Issues
- Integrating cURL into Scripts and Automation
- Best Practices When Using cURL for GET Requests
- Conclusion
Introduction to cURL and GET Requests
cURL (Client for URLs) is a command-line tool and library that facilitates data transfers over various protocols, including HTTP, HTTPS, FTP, and SMTP. Its simplicity and flexibility have made it a favorite for testing APIs, downloading files, and automating tasks.
A GET request is the most common type of HTTP request. It is used to retrieve data from a specific resource without altering anything on the server. This makes GET requests ideal for fetching web pages, images, or API data without causing any side effects. Because GET requests do not modify data, they are safe to repeat multiple times.
Why Use cURL for GET Requests?
cURL’s command-line interface makes it perfect for quick tests, debugging, and integration into scripts or automation workflows. Here are some benefits of using cURL for GET requests:
- Simplicity: A basic GET request can be executed with a single command in your terminal.
- Flexibility: cURL offers a wide range of options (flags) to modify your requests. You can include custom headers, follow redirects, save output to files, and more.
- Portability: cURL is available on almost every operating system, ensuring that your scripts are highly portable.
- Debugging: With options like verbose mode, you can inspect HTTP headers and connection details, making it easier to diagnose issues with your requests.
Anatomy of a cURL GET Request
A typical cURL GET request consists of several components:
- The Command: The
curl
command initiates the tool. - The Method: GET is the default HTTP method, but it can be explicitly specified using the
-X GET
option. - The URL: The target resource you wish to retrieve. In our examples, we use endpoints from the Free Photos API.
- The Options: Various flags modify the request’s behavior (e.g.,
-i
for headers,-o
for output files, and-v
for verbose mode).
A simple GET request might look like this:
curl https://boringapi.com/api/v1/photos/
This command sends a GET request to the specified URL and displays the JSON response directly in your terminal, as shown in the screenshot below:
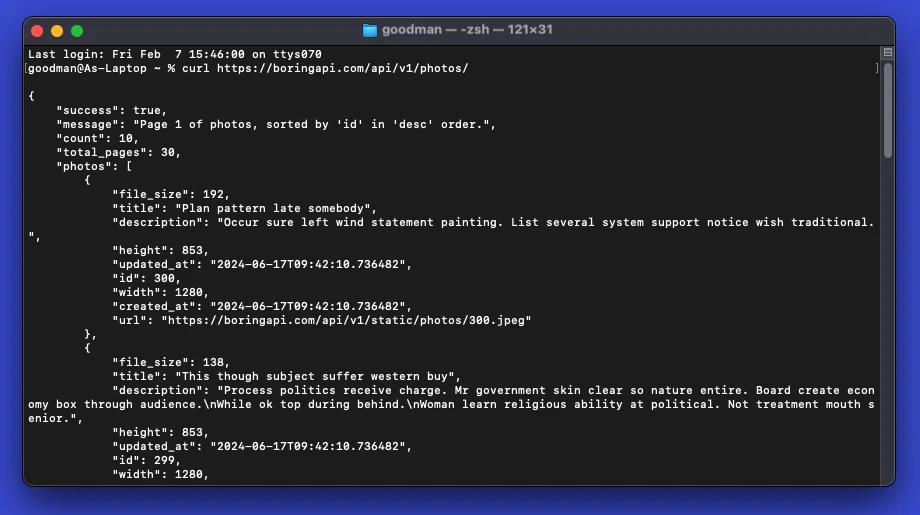
Overview of the Free Photos API
The Free Photos API is an excellent tool for testing and learning how to interact with web APIs. It provides endpoints for:
- Get Photos:
Fetch a list of photos with optional search, pagination, and sorting parameters.
Endpoint:GET https://boringapi.com/api/v1/photos/
Query Parameters:search
: Filter photos by title or description.page
: Specify the page number (default is 1).limit
: Set the number of photos per page (default is 10, min: 1, max: 100).sort_by
: Choose a field to sort by (e.g.,id
,title
).sort_order
: Specifyasc
ordesc
for ascending or descending order.
- Get Random Photos:
Retrieve one or more random photos.
Endpoint:GET https://boringapi.com/api/v1/photos/random
Query Parameter:num
: The number of random photos to return (default is 1, min: 1, max: 10).
- Get a Single Photo:
Fetch a specific photo by its ID.
Endpoint:GET https://boringapi.com/api/v1/photos/{photo_id}
Replace{photo_id}
with the photo’s ID.
Using cURL: Basic Examples
Below are several practical examples that illustrate how to use cURL to interact with the Free Photos API. These examples will help you understand the basics of making GET requests and how to customize them using various options.
Example 1: Basic GET Request for a List of Photos
Open your terminal and run:
curl -X GET "https://boringapi.com/api/v1/photos/"
Output:
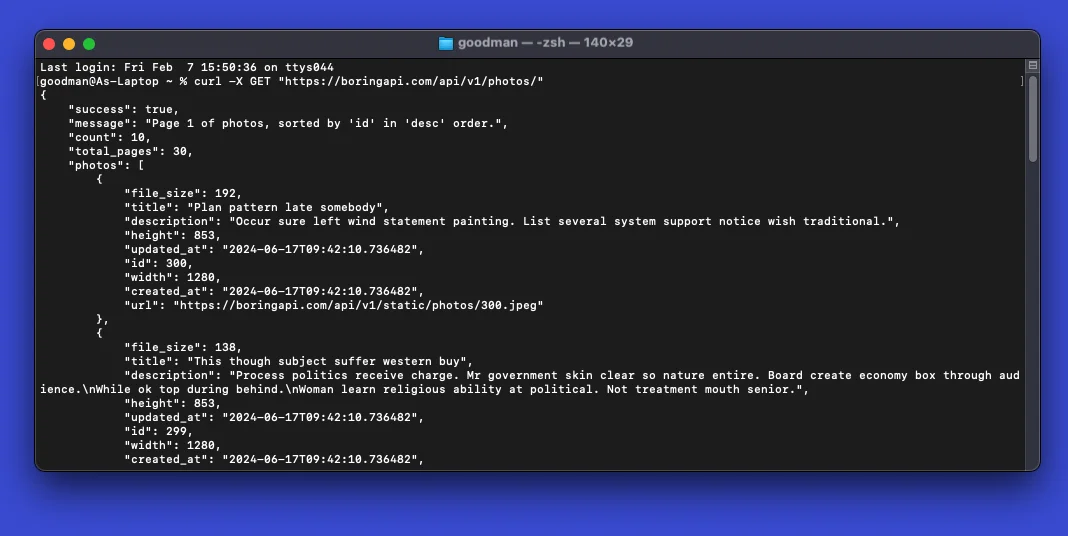
Explanation:
This command sends a GET request to the /photos
endpoint. Without additional parameters, the API returns a default list of photos. The response is in JSON format, including details such as photo IDs, titles, descriptions, dimensions, file sizes, and URLs.
Example 2: GET Request with Query Parameters
To search for photos containing the keyword "somebody" and get the first page of results (up to 10 photos), run:
curl -X GET "https://boringapi.com/api/v1/photos/?search=somebody&page=1&limit=10"
Explanation:
search=somebody
: Filters photos to include only those with "somebody" in their title or description.page=1
: Retrieves the first page of results.limit=10
: Limits the response to 10 photos per page.
This example demonstrates how you can refine your GET request by adding query parameters directly to the URL.
Example 3: GET Request for Random Photos
To fetch random photos, use the /photos/random
endpoint. For example, to get 2 random photos, run:
curl -X GET "https://boringapi.com/api/v1/photos/random?num=2"
Explanation:
The query parameter num=2
specifies that the API should return 2 random photos. The response will include an array of photo objects with their respective details, which is useful for generating dynamic content.
Example 4: GET Request for a Single Photo by ID
To retrieve detailed information about a specific photo, use its unique ID in the URL. For example, to get the photo with ID 1, run:
curl -X GET "https://boringapi.com/api/v1/photos/1"
Explanation:
This command fetches the photo with the specified ID. The returned JSON provides comprehensive details about the photo, such as its title, description, dimensions, file size, and URL.
Advanced cURL Options
cURL offers several advanced options that enhance your GET requests. These options are especially useful for debugging, saving responses, or modifying the request behavior.
Including HTTP Response Headers
To view the HTTP response headers along with the JSON response, add the -i
flag:
curl -i -X GET "https://boringapi.com/api/v1/photos/1"
Result:
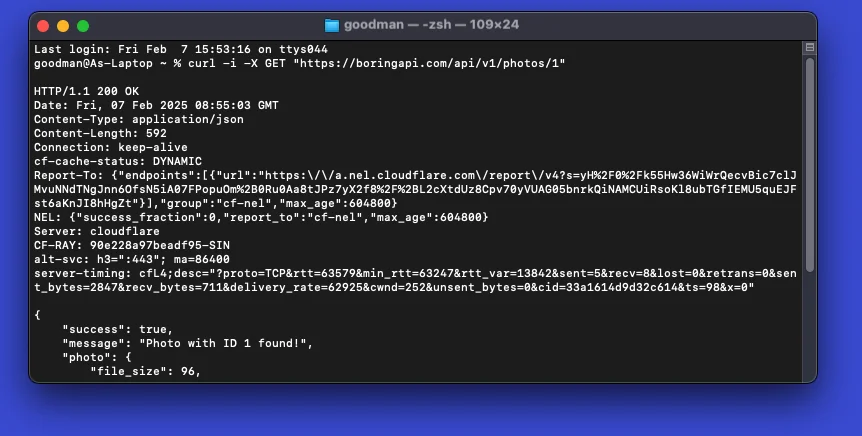
Explanation:
Including headers in your output allows you to inspect the status code, content type, and other metadata. This additional context is valuable for troubleshooting issues and verifying that your request was processed correctly by the server.
Saving Output to a File
If you wish to save the response to a file for further analysis or record keeping, use the -o
flag:
curl -X GET "https://boringapi.com/api/v1/photos/1" -o photo_1.json
Explanation:
This command redirects the JSON output to a file named photo_1.json
instead of displaying it in the terminal. Saving responses is useful when dealing with large data sets or archiving results for later review.
Using Verbose Mode
For detailed debugging information, use the -v
option to enable verbose mode:
curl -v -X GET "https://boringapi.com/api/v1/photos/1"
Explanation:
Verbose mode provides a comprehensive view of the request and response process, including connection details, HTTP headers, and status codes. This information is crucial when diagnosing errors or unexpected behaviors in your API calls.
Setting a Custom User Agent
Some servers may respond differently based on the client’s user agent. To set a custom user agent, use the -A
flag:
curl -A "MyCustomUserAgent/1.0" -X GET "https://boringapi.com/api/v1/photos"
Explanation:
Specifying a custom user agent can help you test how the server handles different clients, bypass certain restrictions, or simply identify your requests in server logs.
Troubleshooting Common Issues
While using cURL for GET requests is generally straightforward, you might encounter some common issues. Here are a few troubleshooting tips:
Connection Timeouts and Network Errors
If you experience connection timeouts or network errors, ensure that your internet connection is stable and that the API server is online. Using verbose mode (-v
) can provide detailed output that may help diagnose connection issues.
Incorrect Query Parameters
If the API response is not what you expected, double-check your query parameters. Ensure that they are spelled correctly and formatted according to the API documentation. Incorrect or misconfigured parameters can lead to unexpected results or error messages.
Response Format Issues
Sometimes, the API might return data in a format you do not expect. To enforce JSON formatting, include the header Accept: application/json
:
curl -H "Accept: application/json" -X GET "https://boringapi.com/api/v1/photos"
Explanation:
This header directs the server to return data in JSON format, which is easier to parse and work with in your applications.
Handling Authentication
Although the Free Photos API does not require authentication, many APIs do. When working with authenticated endpoints, ensure that you use HTTPS and securely pass your credentials (using the -u
flag for basic authentication, for example). Always avoid sending sensitive information in plain text unless absolutely necessary.
Integrating cURL into Scripts and Automation
One of cURL’s key strengths is its seamless integration into scripts. This makes it ideal for automating repetitive tasks and creating complex workflows.
For example, consider a bash script that fetches a list of photos, saves the output to a file, and processes the JSON data with a tool like jq
:
#!/bin/bash
# Fetch a list of photos and save the response to a file
curl -X GET "https://boringapi.com/api/v1/photos?page=1&limit=10" -o photos.json
# Use jq to extract and display the titles of the photos
echo "Photo Titles:"
jq '.photos[].title' photos.json
Explanation:
This script retrieves photo data from the API, saves it to photos.json
, and then uses jq
to parse and print each photo's title. Such automation can be extended to perform more complex data processing or to integrate API responses into larger systems.
Best Practices When Using cURL for GET Requests
To maximize the effectiveness and security of your GET requests, consider the following best practices:
- Read the API Documentation Thoroughly:
Ensure you understand the available endpoints, required parameters, and expected response formats. This prevents errors and helps you construct accurate requests. - Always Use HTTPS:
HTTPS secures your data transmissions, protecting sensitive information and ensuring data integrity. - Test Incrementally:
Begin with simple GET requests and gradually add parameters and options. This incremental approach helps isolate issues when something goes wrong. - Log Your Requests and Responses:
Maintaining logs of your API interactions can be invaluable for debugging and monitoring. Save responses to files if necessary so you can review the raw output later. - Automate Repetitive Tasks:
Integrate cURL commands into scripts to automate regular operations, reducing manual effort and minimizing human error. - Monitor API Changes:
APIs can evolve over time. Regularly review the API documentation for updates and adjust your requests accordingly to maintain compatibility.
Conclusion
cURL is an incredibly powerful and flexible tool for making GET requests from the command line. Although this guide uses the Free Photos API as an example, the techniques and principles discussed here apply to any API or web service. We explored the basics of GET requests, broke down the anatomy of a cURL command, and demonstrated various examples—from fetching lists of photos and filtering results with query parameters to retrieving random photos and obtaining specific photo details by ID.
We also covered advanced cURL options such as including HTTP headers, saving output to a file, enabling verbose mode for debugging, and setting a custom user agent. Additionally, we discussed common troubleshooting strategies and best practices to ensure your API interactions are efficient, reliable, and secure.
By mastering these techniques, you’ll be well-equipped to integrate cURL into your daily workflow—whether you’re testing an API, automating data retrieval, or troubleshooting network issues. With a firm grasp on making GET requests using cURL, you can quickly and efficiently access data from any web service, enhancing your development and operational capabilities.
Happy coding and efficient data fetching with cURL!